We saw in the previous example that it is very easy to use an Edit component to ask the user to input some text, although it must be limited to a single line. In general, it's quite common to ask users for numeric input, too. To accomplish this, you can use the MaskEdit component (in the Additional page of the Components palette) or simply use an Edit component and then convert the input string into an integer, using the standard Pascal Val procedure or the Delphi IntToStr function.
This sounds good, but what if the user types a letter when a number is expected? Of course, these conversion functions return an error code, so we can use it to test whether the user has really entered a number. The second question is, when can we perform this test? Maybe when the value of the edit box changes, when the component loses focus, or when the user clicks on a particular button, such as the OK button in a dialog box. As you'll see, not all of these techniques work well.
There is another, radically different, solution to the problem of allowing only numerical input in an edit box. You can look at the input stream to the edit box and stop any non-numerical input. This technique is not foolproof (a user can always paste some text into an edit box), but it works quite well and is easy to implement. Of course, you can improve it by combining it with one of the other techniques.
The next example, Numbers (see the form in the following figure), shows some of the techniques you can use to handle numerical input with an Edit component, so you can compare them easily. This example is meant as an exercise to discuss keyboard input and the input focus. To handle numerical input in an application you'll generally use specific components, as the SpinEdit or the UpDown controls available in Delphi. We will see an example of the use of another even more sophisticated control for keyboard input, the MaskEdit component.
Intead of pointing to Edit1, we must point to 'the TEdit that generated the event', in other words: the edit-box where the cursor was when a key was depressed. When you look at the template for the event handler that Delphi made, you see the parameter Sender: that's a pointer to the component that generated the event. Professional Notepad is a powerful editor that allows you view and Edit HTML, CSS, JavaScript, PHP, PERL, SQL, Delphi, C and other languages source code.It is a advanced tool supporting the features you've always dreamt about, such as syntax highlighting, Code Templates, bookmarks, unlimited text size, URL highlighting, line numbers, powerful.
In this example we're going to compare the effect of testing the input at different stages. First of all, build a form with five edit fields and five corresponding labels, describing in which occasion the corresponding Edit component checks the input. The form also has a button to check the contents of the first edit field. The contents of the first edit box are checked when the Check button is pressed. In the handler of the OnClick event of this button, the text is first converted into a number, using the Val procedure, which eventually returns an error code. Depending on the value of the code, a message is shown to the user:
procedure TNumbersForm.CheckButtonClick(Sender: TObject); var
Number, Code: Integer; begin if Edit1.Text <> ' then begin
Val (Edit1.Text, Number, Code); if Code <> 0 then begin
Edit1.SetFocus;
MessageDlg ('Not a number in the first edit', mtError, [mbOK], 0);
end else

MessageDlg ('OK, the IntToStr (Number), end; end;
If an error occurs, the application moves the focus back to the edit field before showing the error message to the user, thus inviting the user to correct the value. Of course, in this sample application a user can ignore this suggestion and move to another edit field.
number in the first edit box is' mtInformation, [mbOK], 0);
The same kind of check is made on the second edit field when it loses the focus. In this case, the message is displayed automatically, but only if an error occurs. Why bother the user if everything is fine? The code here differs from that of the first edit field; it makes no reference to the Edit2 component but always refers to the generic Sender control, making a safe typecast. To indicate the number of each button, I've used the Tag property, entering the number of the edit control.
As you can see in the following listing, instead of casting the Sender parameter to the TEdit class several times in the same method, it is better to do this operation once, saving the value in a local variable of the TEdit type. The type checking involved with these casts, in fact, is quite slow.
This method is a little more complex to write, but we will be able to use it again for a different component. Here is its code:
procedure TNumbersForm.Edit2Exit(Sender: TObject); var
Number, Code: Integer; CurrEdit: TEdit; begin
CurrEdit := Sender as TEdit; if CurrEdit.Text <> ' then begin
Val (CurrEdit.Text, Number, Code); if Code <> 0 then begin
CurrEdit.SetFocus;
MessageDlg ('The edit field number ' +
IntToStr (CurrEdit.Tag) + ' does not have a valid number', mtError, [mbOK], 0);
Since Delphi 2, this code produces a warning message (a hint) when compiled, because the Number variable is not used after a value has been assigned to it. To avoid these hints, you can ask the compiler to disable its generation inside a specific method using the $hints compiler directive. Simply write {$hints off} before the method and {$hints on} after it, as I've done in the source code of this example.
The third Edit component makes a similar test each time its content changes (using the OnChange event). Although we have checked the input on different occasions — using different events — the three functions are very similar to each other. The idea is to check the string once the user has entered it.
For the fourth Edit component, I want to show you a completely different technique. We are going to make a check before the Edit even knows that a key has been pressed. The Edit component has an event, OnKeyPress, that corresponds to the action of the user. We can provide a method for this event and test whether the character is a number or the Backspace key (which has a numerical value of 8, so we can refer to it as the character #8). If not, we change the value of the key to the null character (#0), so that it won't be processed by the edit control, and produce a little warning sound: procedure TNumbersForm.Edit4KeyPress(
Sender: TObject; var Key: Char); begin
- check if the key is a number or backspace
- 9', #8]) then if (Key the key in ['0'.
if not begin
The fourth Edit component accepts only numbers for input, but it is not foolproof. A user can copy some text to the Clipboard and paste it into this Edit control with the Shift+Ins key combination (but not using Ctrl+V), avoiding any check. To solve this problem, we might think of adding a check for a change to the contents, as in the third edit field, or a check on the contents when the user leaves the edit field, as in the second component. This is the reason for the fifth, Foolproof edit field: it uses the OnKeyPress event of the fourth edit field, the OnChange method of the third, and the OnExit event of the second, thus requiring no new code.
To reuse an existing method for a new event, just select the Events page of the Object Inspector, move to the component, and instead of double-clicking to the left of the event name, select the button in the combo box at the right. A list of names of old methods compatible with the current event — having the same number of parameters — will be displayed.
If you select the proper methods, the fifth component will combine the features of the third and the fourth. This is possible because in writing these methods, I took care to avoid any reference to the control to which they were related. The technique I used was to refer to the generic Sender parameter and cast it to the proper data type, which in this case was TEdit. As long as you connect a method of this kind to a component of the same kind, no problem should arise. Otherwise, you should make a number of type checks (using the is operator), which will probably make the code more complex to read. My suggestion is to share code only between controls of the same kind.
Notice also that to tell the user which edit box has incorrect text, I've added to each Edit component a value for the Tag property, as I mentioned before. Every edit box has a tag with its number, from 1 to 5.
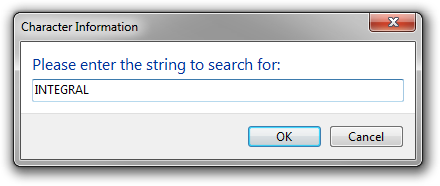
Continue reading here: Sophisticated Input Schemes
Was this article helpful?
- 3Uses
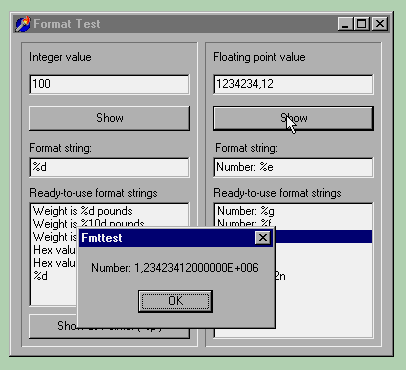
Delphi Numeric Edit Box Codes
The following example demostrates the use of some advancedmethod in the Character unit. An edit box is configuredto allow 2 unicode characters (since there may be surrogate pairs).
Delphi Numeric Edit Box Code Free
- System.Character.IsLetterOrDigit( fr | de | ja )
- System.Character.IsControl( fr | de | ja )
- System.Character.ConvertFromUtf32( fr | de | ja )
- System.Character.GetNumericValue( fr | de | ja )
- System.Character.GetUnicodeCategory( fr | de | ja )
- System.Character.TUnicodeCategory( fr | de | ja )
- Vcl.StdCtrls.TCustomEdit.MaxLength( fr | de | ja )